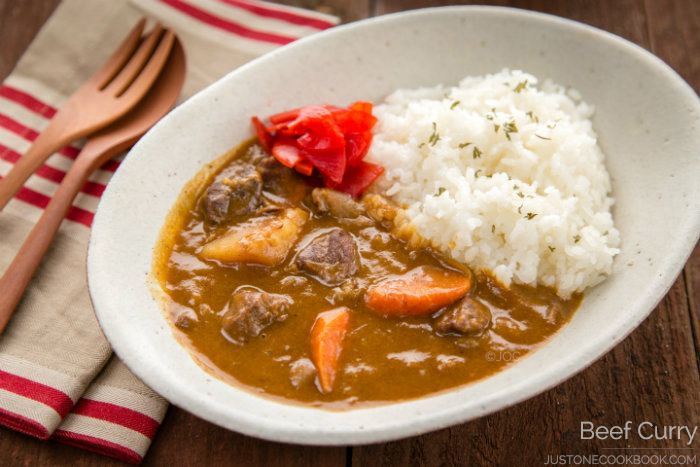
There are a lot of really technical definitions and lots of great information out there about function currying, but if you haven't taken classes on algorithms or don't have a Math or CS degree, it can seem pretty confusing. Function Currying is creating a function that returns another function with parameters set from the first function. Ok, not so simple in words, but it's not that complex. For an exact definition of it feel free to read up on it at Wikipedia, but since it's easier to just look at some code and see how it works, let's just take a look and see exactly what it is.
The Basic Example

On line 7 however, we have something that looks a bit different. This is an example of function currying. What is happening is that the function addCurried isn't returning a value, it's returning an entire function, and creating a scope, with Y set to the value used in the function. On line 15 you can see an example of it being used to create a function called add_5 with the Y value in the function returned set to 5. Then that function can be used, and it will work just like the add function, except that you only pass in the X value, because the Y is always set to 5. You can also call it like addCurried(5)(3) to get 8 as well since addCurried returns a function which can then be called with the parameter (3).
If you don't know a ton about scope and closures in JavaScript and you're thinking: How can this all be so?! Go read You Don't Know JS - Scopes and Closures. It will really take your understanding of JavaScript scopes to a new level (and it's free online). Function currying isn't that scary at all, it's just a way to create a function with "preset values" so that you can reuse parts of a function. This becomes extremely useful when building callback functions and helps keep your code DRY.
Practical Usage
There are TONS of uses for function currying, but one that I often employ is using function currying to create middleware or callbacks that can be modified for multiple functions.
For example, say you're using Express and you want to authenticate a user token against different scopes for different endpoints. Middleware makes that super easy, but you could spend a lot of time with anonymous functions doing logic for each endpoint if you don't keep your code dry.
First let's take a look at a very basic section of code without function currying. In this section of code below, you can see that I made 2 routes, and in each route, I'm checking a req.decoded_token.scopes value against a list of scopes required for this endpoint. If the validation fails, the response redirects to another endpoint and doesn't allow the user to pass.
This works, but is messy and isn't very extensible, nor is it DRY at all. An easy way to make this code look a lot nicer, is to use function currying, or to be more precise, partial function currying. Since we have 2 values that change from endpoint to endpoint: the scopes to validate against, and the endpoint to redirect to, we can create a middleware creator to curry those values onto a common logic base.
With this second iteration of the code, you can see it looks a LOT nicer and certainly more DRY. The function createMiddleware takes 2 parameters, our scopes we want to validate against, and the endpoint we want to redirect to, and returns a function which will be used as the middleware function for our application endpoints.
Function Currying and Partial Currying can be extremely useful in JavaScript particularly when dealing with anonymous functions or callbacks, and I often employ it to build handlers and helpers that can create the anonymous functions I need while being flexible and letting me specify the parameters.
I hope these snippets of code were some examples of how you can use currying to keep your code DRY. It is a really powerful skill to learn in JavaScript and having a good handle on how to use it properly will allow you to save a lot of time and effort.
No comments:
Post a Comment